Filter an array in CoffeeScript: CoffeeScript, known for its elegant syntax and enhanced readability, simplifies many tasks that might be more verbose in other programming languages. One common operation developers often encounter is filtering arrays. In this article, we’ll explore various techniques to filter an array in CoffeeScript and dive into frequently asked questions to help you master this essential skill.
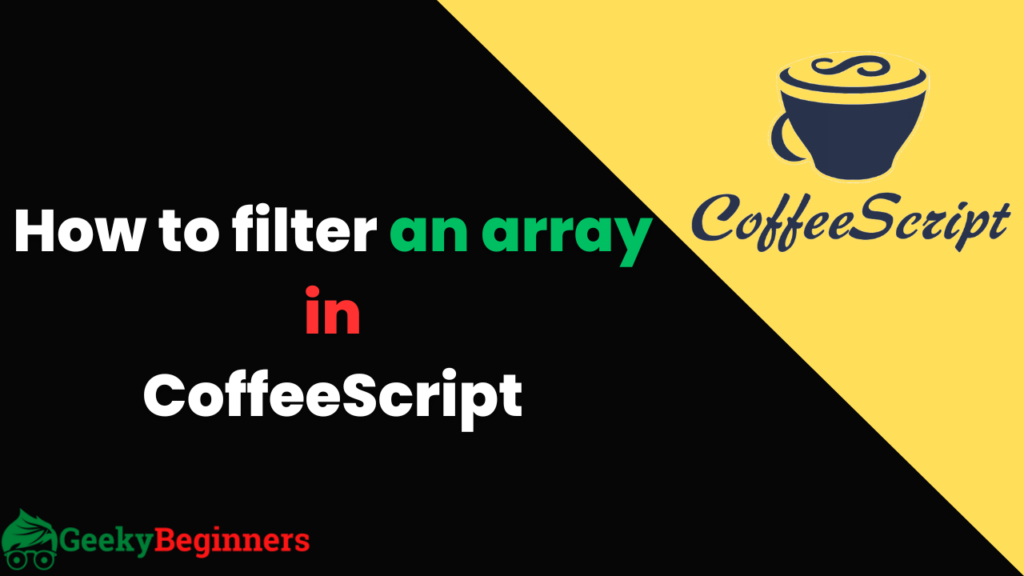
Table of Contents
Basics of Array Filtering in CoffeeScript
Using the filter
Function
One of the simplest ways to filter an array in CoffeeScript is by using the built-in filter
function. This higher-order function takes a callback that specifies the filtering condition. Let’s take a look at a basic example:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] # Filtering even numbers evenNumbers = numbers.filter((num) -> num % 2 == 0 ) console.log(evenNumbers)
Array Comprehension
CoffeeScript provides a concise syntax called array comprehension for creating new arrays. It’s another effective way to filter arrays:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] # Filtering even numbers evenNumbers = (num for num in numbers when num % 2 == 0) console.log(evenNumbers)
Advanced Filtering Techniques
Chaining Filters
CoffeeScript allows you to chain multiple filters together, providing a clean and readable way to apply complex conditions:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] # Filtering numbers divisible by 3 and 5 filteredNumbers = numbers.filter((num) -> num % 3 == 0 ).filter((num) -> num % 5 == 0 ) console.log(filteredNumbers)
Leveraging CoffeeScript’s Expressive Syntax
CoffeeScript’s expressive syntax enables concise yet clear filtering conditions. Utilize this feature to make your code more readable:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] # Filtering odd numbers using a concise condition oddNumbers = numbers.filter((num) -> num % 2 != 0) console.log(oddNumbers)
Conclusion
Filtering arrays in CoffeeScript is a fundamental skill that enhances the readability and maintainability of your code. Whether you prefer the expressive power of the filter
function or the concise syntax of array comprehensions, mastering these techniques will empower you to efficiently manipulate arrays in your CoffeeScript projects.