Export default in JavaScript: JavaScript, as one of the most popular programming languages, offers a versatile module system for organizing and structuring code. Among the various ways to export and import modules in JavaScript, the export default
statement plays a pivotal role. In this article, we will delve into the complications of using export default
to enhance your understanding of modular JavaScript programming.
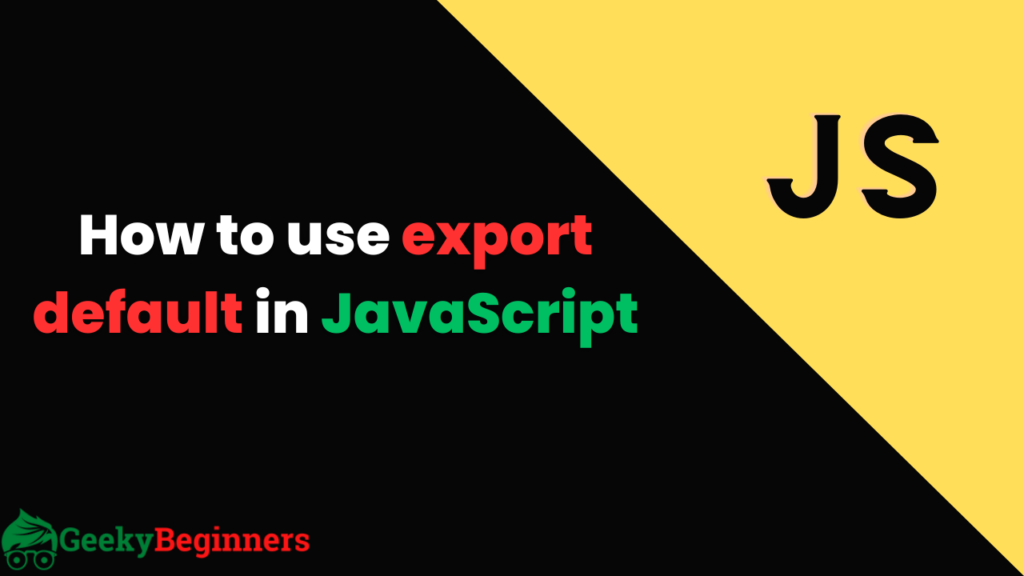
Table of Contents
What are Modules in JavaScript
Before we explore export default
, let’s have a quick refresher on JavaScript modules. Modules are a way to organize code into reusable files, making it easier to maintain and scale applications. In JavaScript, the import
and export
statements facilitate the modular structure, allowing us to break down our code into smaller, manageable pieces.
What is Export in JavaScript?
The export
statement is used to expose functionalities, variables, or objects from a module, making them accessible to other parts of your code. For example, consider the following module named myModule.js
:
// myModule.js export const myVariable = 42; export function myFunction() { // Function logic here }
In another file, you can import the exported elements like this:
import { myVariable, myFunction } from './myModule.js'; console.log(myVariable); // Output: 42 myFunction();
How to use export default in JavaScript
Now, let’s focus on export default
. Unlike the export
statement, which allows you to export multiple entities from a module, export default
is used to export a single value, function, or object as the default export from a module. This means that when you import the module, the default export is what you get if you don’t specify a particular import.
Here’s an example to illustrate the concept:
// myDefaultModule.js const defaultExport = 10; export default defaultExport;
In another file, you can import the default export like this:
import myDefaultExport from './myDefaultModule.js'; console.log(myDefaultExport); // Output: 10
Key Points to remember when using export default in JavaScript
- Only one default export per module: Unlike
export
, which allows multiple exports, a module can have only one default export. This makes it clear and concise when working with default exports. - No curly braces for default imports: When importing the default export, you don’t use curly braces. Instead, you directly assign the imported value to a variable, as shown in the example above.
- Default exports can have any name: While it’s a common convention to name the default export as
default
, you can actually name it anything you want. However, usingdefault
enhances code readability.
Conclusion
In conclusion, export default
is a powerful feature in JavaScript that allows you to export a single value, function, or object as the default export from a module. This provides a clean and intuitive way to structure your code, making it more readable and maintainable. By understanding the basics of export default
, you can leverage this feature to build modular and scalable JavaScript applications.
- Also Read: How to generate dates between two dates in JavaScript
- Also Read: How to create resend otp timer using HTML/CSS and JavaScript