Dynamically Filtering an Array of Objects in JavaScript: JavaScript is a versatile programming language widely used for building dynamic web applications. One common task in web development is manipulating and filtering data, especially when working with arrays of objects. Dynamically filtering an array of objects allows developers to display relevant information to users based on specific criteria. In this article, we’ll explore various methods to achieve dynamic filtering in JavaScript.
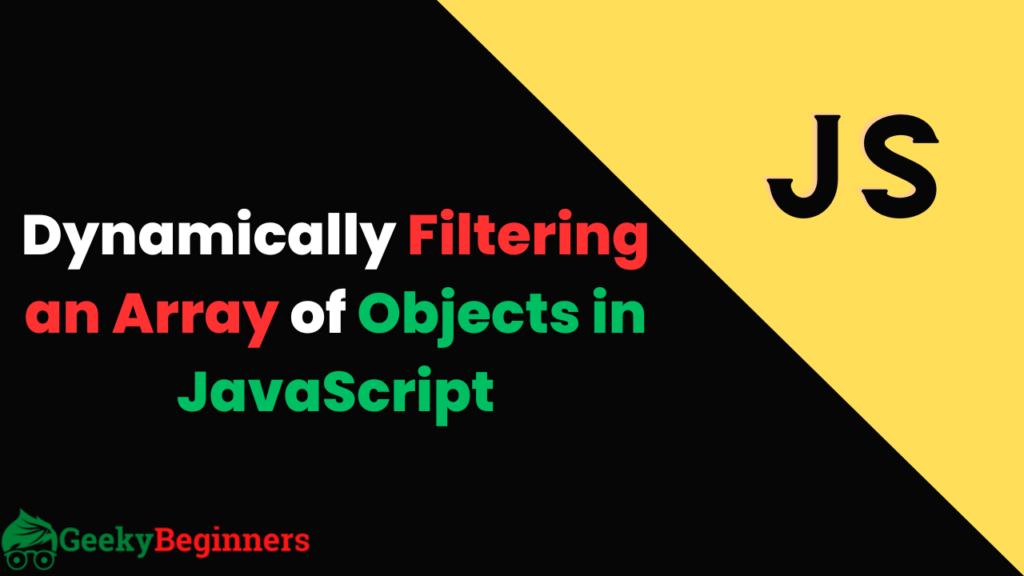
Table of Contents
What is Array of Objects
Before diving into dynamic filtering, let’s briefly understand arrays of objects. An array of objects is a collection of objects where each object contains key-value pairs. For example:
const students = [ { id: 1, name: 'Alice', age: 22, grade: 'A' }, { id: 2, name: 'Bob', age: 25, grade: 'B' }, { id: 3, name: 'Charlie', age: 21, grade: 'C' }, // ... more objects ];
Each object in the array represents a student with properties like id
, name
, age
, and grade
.
Static Filtering in JavaScript
Static filtering involves hardcoding the filtering criteria. For example, to filter students older than 21:
const filteredStudents = students.filter(student => student.age > 21);
However, dynamic filtering implies that the filtering criteria are not known beforehand and can change based on user input or other factors.
Dynamically Filtering an Array of Objects in JavaScript
Filter Method
The filter
method is a powerful tool for dynamically filtering arrays. It creates a new array with elements that pass the given test. We can dynamically define the filtering logic:
function dynamicFilter(array, filterCriteria) { return array.filter(object => { // Implement dynamic filtering logic based on filterCriteria // ... }); } // Example usage const ageFilter = 21; const filteredStudentsByAge = dynamicFilter(students, student => student.age > ageFilter);
This method allows for flexible and reusable filtering logic.
Object Property as a Filter
You can use an object to represent the filter criteria, making it easy to adjust dynamically:
function dynamicFilterWithObject(array, filterObject) { return array.filter(object => { for (const key in filterObject) { if (object[key] !== filterObject[key]) { return false; } } return true; }); } // Example usage const ageFilter = 21; const gradeFilter = 'A'; const filteredStudents = dynamicFilterWithObject(students, { age: ageFilter, grade: gradeFilter });
This approach is extensible and accommodates multiple filter criteria.
Using Function Composition
Function composition involves combining functions to create a more complex function. In the context of dynamic filtering, this allows for the creation of modular and reusable filter functions:
function ageFilter(age) { return student => student.age > age; } function gradeFilter(grade) { return student => student.grade === grade; } const combinedFilter = (filters) => (item) => filters.every(filter => filter(item)); // Example usage const ageCriteria = ageFilter(21); const gradeCriteria = gradeFilter('A'); const filteredStudents = students.filter(combinedFilter([ageCriteria, gradeCriteria]));
This approach is particularly useful when dealing with complex filtering requirements.
Conclusion
Dynamically filtering an array of objects in JavaScript is a crucial skill for web developers. Whether you choose the filter
method, object-based filtering, or function composition, the key is to create flexible, reusable, and modular code. This ensures that your application can efficiently adapt to changing requirements and user interactions. Experiment with these methods and choose the one that best fits your specific use case.
- How to use export default in JavaScript
- How to generate dates between two dates in JavaScript
- How to create resend otp timer using HTML/CSS and JavaScript