In this article, we will see what is the “Uncaught TypeError: cannot set property innerHTML of null” error and what are the possible ways to fix the error easily. This error occurs when JavaScript fails to set the innerHTML property to a null value. In this post, we will see how we can fix the error.
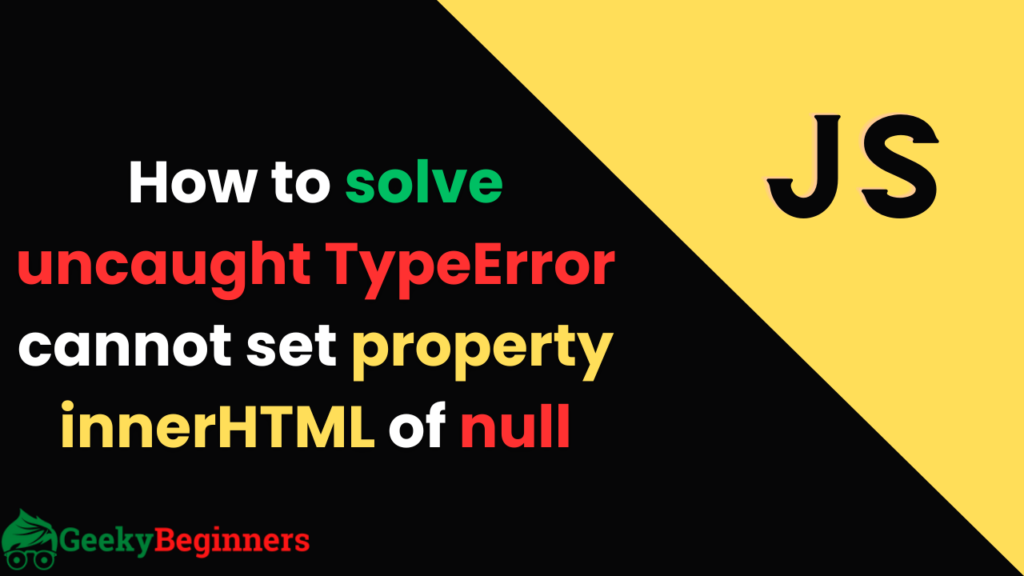
Table of Contents
Uncaught TypeError cannot set property innerHTML of null
This error occur when JS is not able to set the property of innerHTML to a null value. Have a look at some examples below to get a proper understanding of the error.
<!DOCTYPE HTML> <html> <head> <title>Uncaught TypeError</title> <script> setValue(); function setValue(){ document.getElementById('my_div').innerHTML = 'JS Course'; }; </script> </head> <body> <div id="my_div"></div> </body> </html>
This code will throw an error “Uncaught TypeError: cannot set property innerHTML of null”. As we are writing JS code before the div element. Our JS code is trying to set the innerHTML property by ID which is written after the JS code. So this is unable to fine the div tag as code flows from top to bottom, and this will throw an error.
How to solve uncaught TypeError cannot set property innerHTML of null
There are various methods to solve the error “Uncaught TypeError: cannot set property innerHTML of null” but we are going to explain here to fix this error.
- Define div Element before the JavaScript code.
- Using window.onload function to execute script at the very last.
- Using the ready() function of the JQuery library.
Define div Element before the JavaScript code
The simplest way to fix this error is just define all the div elements for which you want to use script before the JS script. See the fix in below code:
<!DOCTYPE HTML> <html> <head> <title>Uncaught TypeError</title> </head> <body> <div id="my_div"></div> //Placing Script After div <script> setValue(); function setValue(){ document.getElementById('my_div').innerHTML = 'JS Course'; }; </script> </body> </html>
Note: It is not a good programming practice to use the script inside the body tag.
Using window.onload function to execute script at the very last
We can use window.onload function to execute our JS script after the whole page is loaded this will not let you use the bad programming practice of using script tag inside the body tag. Window.onload functions let your JavaScript script wait for the entire page to load first and then the JavaScript will execute.
<!DOCTYPE HTML> <html> <head> <title>Uncaught TypeError: innerHTML to null</title> <script> function setValue(){ document.getElementById('my_div').innerHTML = 'JS Course'; } window.onload = function () { setValue(); } </script> </head> <body> <div id="my_div"></div> </body> </html>
In this example code, we used windows.onload function to execute our script as soon as the page loads. We define the script tag inside the head tag which is a good practice.
Using the ready() function of the JQuery library
We can use the ready() function instead of window.onload, working of both the function is same. So to use the ready() function we need to first include Jquery library. See the code below:
<!DOCTYPE HTML> <html> <head> <title>Uncaught TypeError: innerHTML to null</title> <script src="https://code.jquery.com/jquery-1.9.1.min.js"></script> <script> function setValue(){ document.getElementById('my_div').innerHTML = 'JS Course'; } $( document ).ready(function() { setValue(); }); </script> </head> <body> <div id="my_div"></div> </body> </html>
In this example code, we are including Jquery Library using the script tag. This library contains the ready() function which is used to execute the script after the whole page loads. Here in the code setValue() function will be called after the page will be loaded.
Conclusion
So we have discussed 3 ways here by using them you will be able to fix the error “Uncaught TypeError: cannot set property innerHTML of null”. We would recommend using 2nd method which is by using window.onload function because using this will not require headache to include any library also we do not need to take care to use the script tag at the bottom of the body tag which is also not a good practice.