TypeError: Failed to Fetch error may occur in JavaScript when you work with the Fetch API, which is used for making network requests.
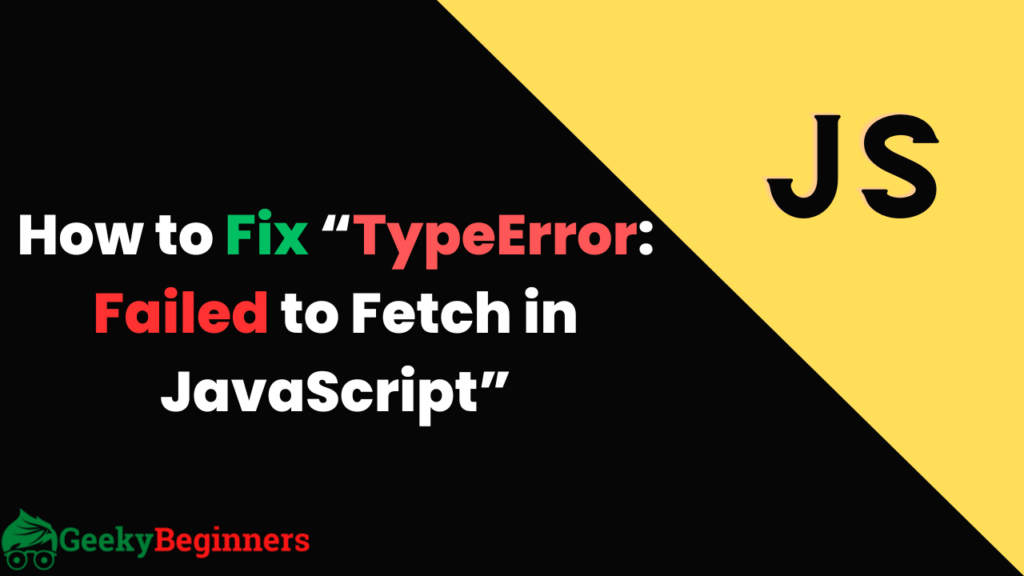
JavaScript plays a crucial role in web development, enabling dynamic and interactive features on websites. However, encountering errors is inevitable, and one common issue developers face is the “TypeError: Failed to Fetch.” This error occurs when the fetch
API, used for making network requests, encounters a problem. In this article, we’ll explore the potential causes of this error and provide solutions to help you troubleshoot and fix it.
Table of Contents
Understanding the Error TypeError: Failed to Fetch
Before diving into solutions, it’s essential to understand the nature of the error. The “TypeError: Failed to Fetch” typically appears in the browser’s console when a request made using the fetch
function encounters an issue, such as network problems, server-side errors, or incorrect API usage.
Common reasons causing the error TypeError: Failed to Fetch
There are several reasons which can cause this error, so lets discuss them one by one.
Network Issues
The server could be inaccessible, or there might be other network-related issues hindering the successful completion of the fetch request.
CORS (Cross-Origin Resource Sharing) Policy
Encountering CORS problems is a possibility when attempting to request a domain different from the hosting domain of your site. For successful communication, the server you are reaching out to must incorporate the necessary CORS headers in its response; without them, browsers will prohibit the request as a security precaution.
Server-Side Errors
There could be a possible scenario where server might not support the HTTP method you’re using in your fetch request.
Incorrect API Usage
The “Incorrect API Usage” point in the article refers to situations where the usage of the Fetch API in JavaScript may not align with the expected or correct syntax, resulting in the “TypeError: Failed to Fetch” error.
Mixed Content Issues (HTTPS/HTTP)
When attempting to initiate requests from an HTTPS site to an HTTP endpoint, browsers may restrict it due to mixed content limitations.
Solutions to Fix “TypeError: Failed to Fetch”
Check Network Connection
- Verify that your internet connection is stable.
- Ensure that the server you are trying to reach is up and running.
Handle Fetch Errors
- Implement error handling in your
fetch
calls to catch and log any issues.
fetch('https://example.com/api/data') .then(response => { if (!response.ok) { throw new Error('Network response was not ok'); } return response.json(); }) .catch(error => console.error('Fetch Error:', error));
Configure CORS Headers
- Set up appropriate CORS headers on the server.
- Allow specific domains to access the server’s resources.
Use HTTPS
- Ensure that your site and API endpoints use HTTPS.
- Modern browsers may block requests from secure sites to non-secure (HTTP) endpoints.
Conclusion
The “TypeError: Failed to Fetch” in JavaScript can be caused by various factors, ranging from network issues to server-side problems. By understanding the common causes and applying the suggested solutions, you can effectively troubleshoot and fix this error in your web applications. Remember to handle errors gracefully, provide meaningful error messages, and regularly test your applications to ensure a smooth user experience.