Generate dates between two dates in JavaScript: Hey Folks! In this tutorial, we will understand how to generate dates between two dates in JavaScript. There are certain cases when we need to find out all dates between two given dates. e.g let’s say we have given a date and we need to find the date 3 days after the given date or 400 days after the given date so in such scenarios we need to find dates in between to get the desired result. Let’s understand the concept by some examples:
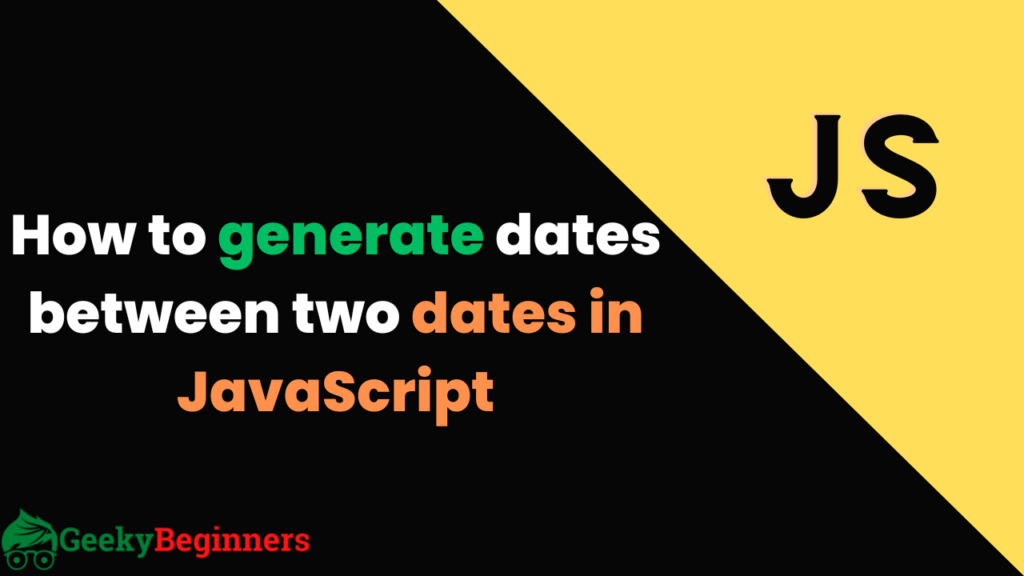
Table of Contents
JavaScript Code to generate dates between two dates in JavaScript
function getDatesBetween(startDate, endDate) { const currentDate = new Date(startDate.getTime()); const dates = []; while (currentDate <= endDate) { dates.push(new Date(currentDate)); currentDate.setDate(currentDate.getDate() + 1); } return dates; } const date1 = new Date('2022-04-15'); const date2 = new Date('2022-04-30'); allDates = getDatesBetween(date1, date2); console.log(allDates);
Output
[ 2022-04-15T00:00:00.000Z, 2022-04-16T00:00:00.000Z, 2022-04-17T00:00:00.000Z, 2022-04-18T00:00:00.000Z, 2022-04-19T00:00:00.000Z, 2022-04-20T00:00:00.000Z, 2022-04-21T00:00:00.000Z, 2022-04-22T00:00:00.000Z, 2022-04-23T00:00:00.000Z, 2022-04-24T00:00:00.000Z, 2022-04-25T00:00:00.000Z, 2022-04-26T00:00:00.000Z, 2022-04-27T00:00:00.000Z, 2022-04-28T00:00:00.000Z, 2022-04-29T00:00:00.000Z, 2022-04-30T00:00:00.000Z ]
Explanation
In this illustration, we’ve crafted a function named getDatesBetween()
that takes two parameters, namely startDate
and endDate
. Sandwiched between these two dates are a bunch of dates we want to unveil. So, the mission here is to print out all those dates.
The action kicks off by creating a date object from the startDate
, neatly stashing it in a shiny new variable called currentDate
. Simultaneously, we’ve birthed a team of dates, corralled within an array aptly named ‘dates.’ Now, the real magic happens within a trusty while loop. The condition is simple: as long as currentDate
remains less than or equal to endDate
, we keep the loop churning.
With each iteration, the currentDate
earns its spot in the ‘dates’ array. A quick date incrementation by 1 (currentDate.setDate(currentDate.getDate() + 1)
) ensures the progression through this chronological quest. The loop tirelessly executes until we reach the endDate
, at which point the function proudly delivers the populated ‘dates’ array.
To wrap up this date extravaganza, we unfurl the dates using the trusty console.log()
function. Voilà!
Conclusion
In the scenario presented here, both the startDate
and endDate
are part of the final list of dates. However, should you wish to omit the endDate
from this list, be sure to tweak the while condition from (currentDate <= endDate)
to (currentDate < endDate)
. Additionally, switch the positions of the two lines within the while loop accordingly.