Resend OTP Timer: In many web applications, the use of OTPs (One-Time Passwords) is crucial for enhancing security. To provide a seamless user experience, it’s essential to implement a feature that allows users to resend the OTP in case they didn’t receive it or it expired. In this tutorial, we’ll guide you through the process of creating a resend OTP timer using HTML, CSS, and JavaScript.
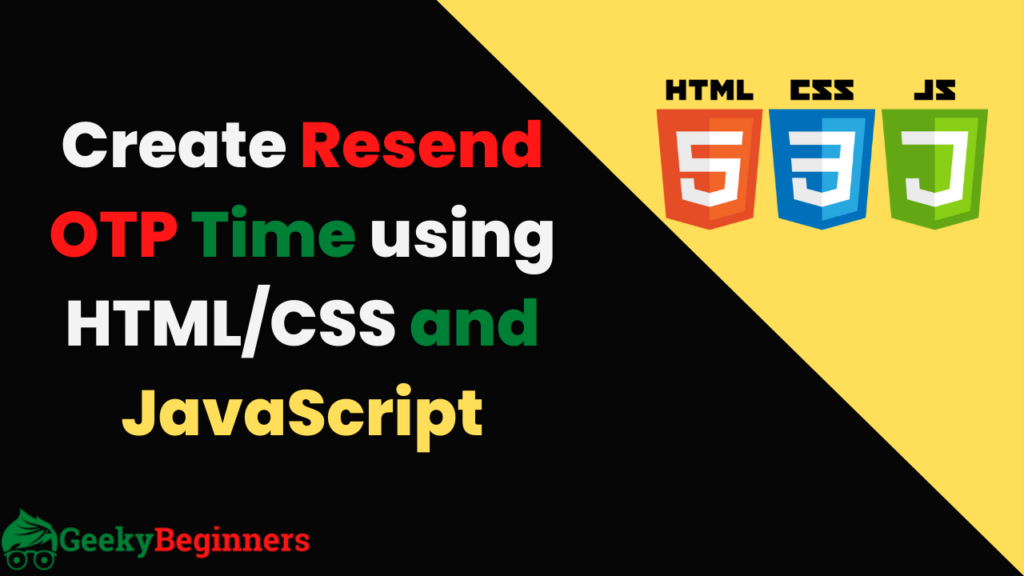
Table of Contents
Create Resend OTP Timer
To create resend otp timer using HTML/CSS and JavaScript, we should have basic understanding of HTML and JavaScript so that you can modify the code as per your need. Don’t worry If you don’t know about it you can just use the code as is.
Setting Up HTML Structure
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Resend OTP Timer</title> <link rel="stylesheet" href="styles.css"> </head> <body> <div class="otp-container"> <input type="text" id="otpInput" placeholder="Enter OTP"> <button id="resendBtn" onclick="startResendTimer()">Resend OTP</button> <p id="timer"></p> </div> <script src="script.js"></script> </body> </html>
Resend OTP Timer Styling With CSS
Create a file named styles.css
and add the following styles:
body { font-family: 'Arial', sans-serif; display: flex; align-items: center; justify-content: center; height: 100vh; margin: 0; } .otp-container { text-align: center; } input { padding: 10px; margin: 10px; } button { padding: 10px; margin: 10px; cursor: pointer; } #timer { font-size: 18px; }
Implementing JavaScript Functionality
Create a file named script.js
and add the following JavaScript code:
let timer; let countdown = 60; // Set the countdown duration in seconds function startResendTimer() { // Disable the button during the countdown document.getElementById('resendBtn').disabled = true; // Start the countdown timer = setInterval(updateTimer, 1000); } function updateTimer() { const timerElement = document.getElementById('timer'); if (countdown > 0) { timerElement.textContent = `Resend in ${countdown} seconds`; countdown--; } else { // Enable the button when the countdown reaches zero document.getElementById('resendBtn').disabled = false; timerElement.textContent = ''; // Reset countdown for the next attempt countdown = 60; // Stop the timer clearInterval(timer); } }
Testing Resend OTP Timer
Open the HTML file in a web browser, and you should see a simple OTP input field, a “Resend OTP” button, and a timer. Clicking the “Resend OTP” button will start the countdown, and the button will be disabled during this time.
- Also Read: Currency Converter in JavaScript
Demo of Resend OTP Timer using HTML/CSS and JavaScript
Below is the preview created in codepen you can copy paste the code and make changes according to your need.
See the Pen Resend OTP Timer by Diwakar Blogging (@Diwakar-Blogging) on CodePen.
Conclusion
Congratulations! You’ve successfully created a resend OTP timer using HTML, CSS, and JavaScript. Feel free to customize the styles or adjust the countdown duration according to your application’s requirements.