Merge Arrays without duplicates in JavaScript: Many times we come up to a situation where we need to merge two arrays and remove the duplicate values from the resulting array. This could be a challenging task for someone. In this article we will show you how you can merge arrays without duplicates in JavaScript.
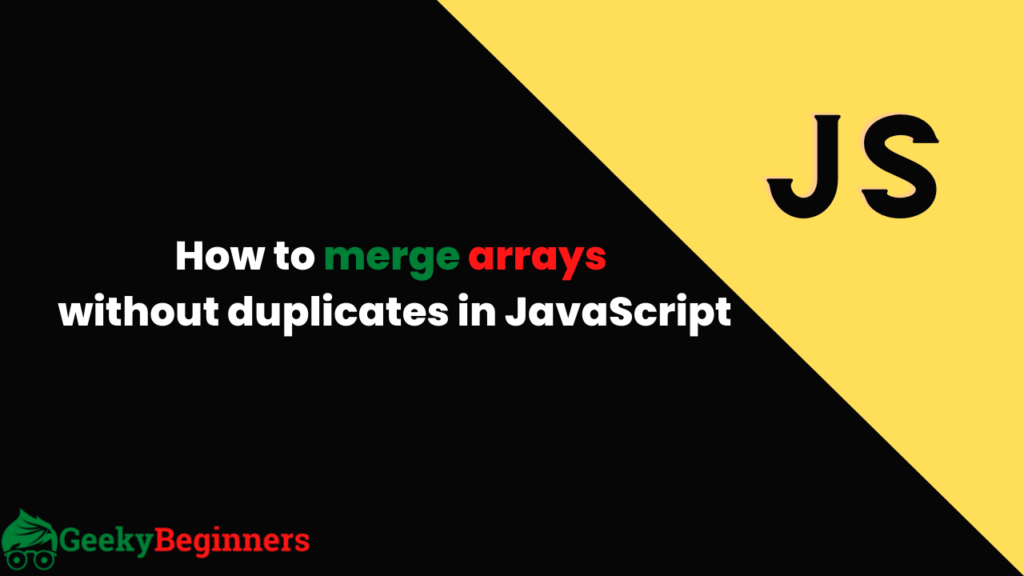
We are discussing 3 ways to merge arrays without duplicates in JavaScript.
- Using concat() and for loop
- Using concat() and filter (ES5 Solution)
- Using Spread Syntax and Set (ES6 Solution)
Table of Contents
Using concat() and for loop
concat() function in JavaScript is used to merge two arrays. But only concat() function can’t prevent duplicates so we have to loop it and push only unique elements in the resulting array.
Example Source Code:
// program to merge and remove duplicate value from an array function mergeUniqueArray(arr1, arr2){ // merge two arrays let arr = arr1.concat(arr2); let uniqueArray = []; // loop through array for(let i of arr) { if(uniqueArray.indexOf(i) === -1) { uniqueArray.push(i); } } console.log(uniqueArray); } const array1 = [1, 2, 3, 4, 5]; const array2 = [3, 4, 5, 6]; // calling the function // passing array argument mergeUniqueArray(array1, array2);
Output:
[1, 2, 3, 4, 5, 6]
Explanation:
In the example, above we are merging two arrays using concat() function and by using for loop we are only pushing the unique values in the new merged array.
Here,
- The two arrays are merged using the
concat()
method. - The
for...of
loop is used to loop through all the elements of arr. - The
indexOf()
method returns -1 if the element is not in the array.
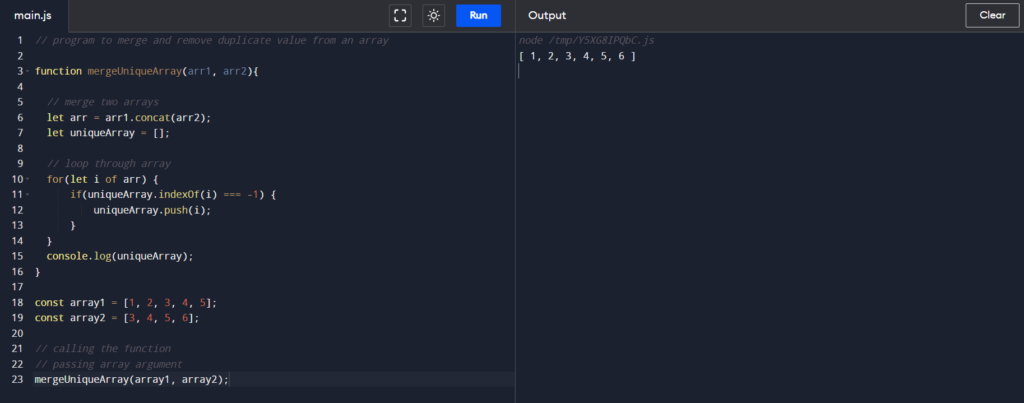
Using concat() and filter (ES5 Solution)
ES5 added a new function named filter() function. We can now use filter() function with concat() to get the merged arrays without any duplication.
Example Source Code:
// program to merge and remove duplicate value from an array let array1 = ['a','b','c'] let array2 = ['c','c','d','e', 'f']; // merge two arrays let mergedUniqueArray = array1.concat(array2); mergedUniqueArray = mergedUniqueArray.filter((item,index)=>{ return (mergedUniqueArray.indexOf(item) == index) }) console.log(mergedUniqueArray);
Output:
[ 'a', 'b', 'c', 'd', 'e', 'f' ]
Explanation:
In the example above, We declared two arrays containing some alphabets. Then we are merging them using concat() function after that we have written a filter function to filter unique values and assign them to the resulting array. Finally, we are printing the resulting array by using console.log() function.
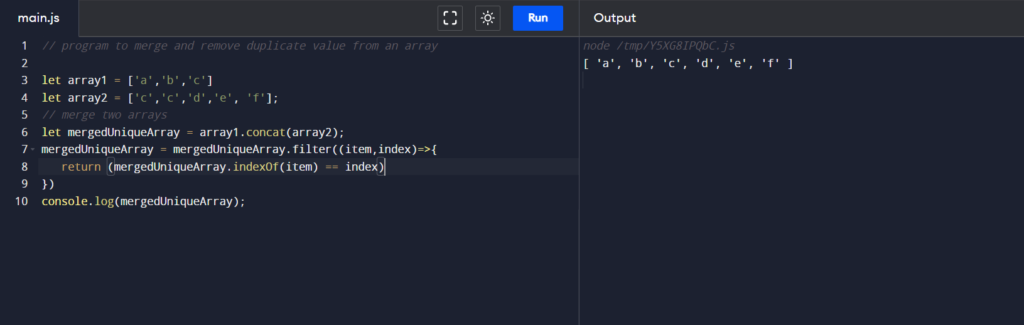
Using Spread Syntax and Set (ES6 Solution)
ES6 introduced Spread syntax(…) which can be used when all elements from an object or array need to be included in a list of some kind.
Example Source Code:
// program to merge and remove duplicate value from an array function mergedUniqueArray(arr1, arr2){ // merge two arrays let mergedArray = [...arr1, ...arr2]; // removing duplicate let uniqueArray = [...new Set(mergedArray)]; console.log(uniqueArray); } const array1 = [1, 2, 3, 4, 5]; const array2 = [3, 4, 5, 6]; // calling the function mergedUniqueArray(array1, array2);
Output:
[1, 2, 3, 4, 5, 6]
Explanation:
In the above example program, two arrays are merged together using spread syntax (...
) and Set
is used to remove duplicate items from resulting array.
We know that The Set
is a collection of unique values.
Here,
- Two array elements are merged together using the spread syntax
...
- The array is converted to
Set
and all the duplicate elements are automatically removed. - The spread syntax
...
is then used to include all the elements of the set back to an array.
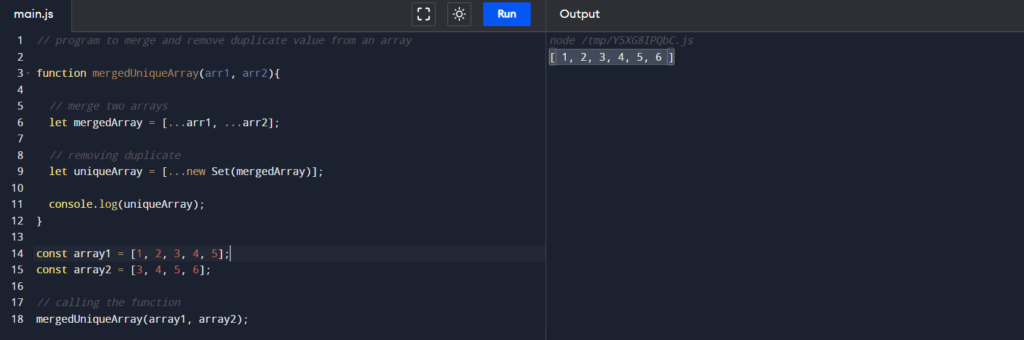
Conclusion
So these are some standard ways to merge arrays without duplicates in JavaScript. But the solution provided by ES6, is the simplest and fast one. With a time complexity of O(n), this is the most ideal choice for any merging two, or more arrays without duplicates in JavaScript. Thanks for reading our article, I hope that you found it helpful! if yes make sure to comment.