How to make footer stick to bottom in React: Creating a sleek and user-friendly website often involves paying attention to the finer details, and one such detail is the footer. While footers provide valuable information and navigation links, ensuring they stay at the bottom of the page can be a bit tricky, especially when working with React. Fear not! In this guide, we’ll explain making your footer stick to the bottom in React, giving your website that polished and professional touch.
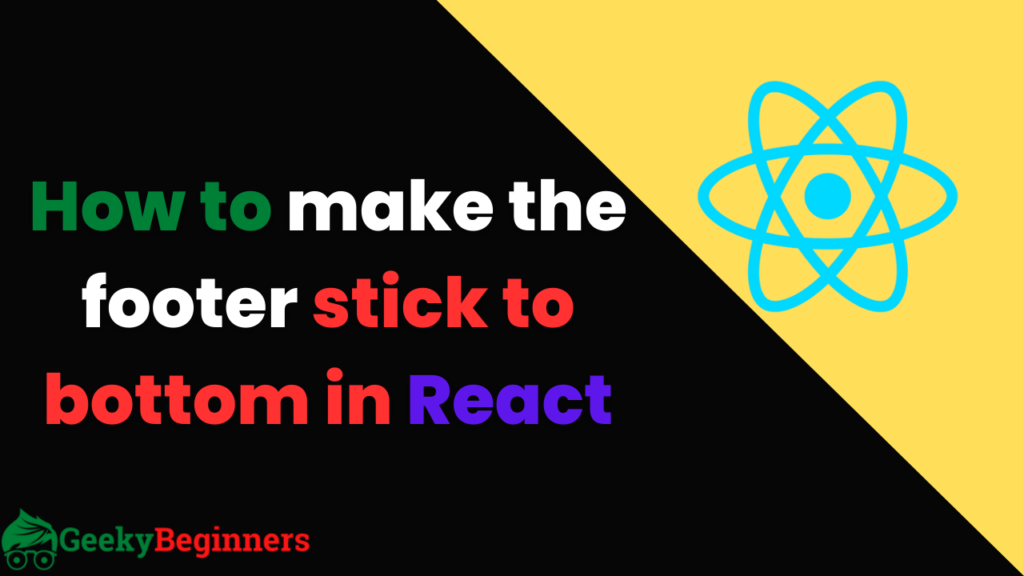
In the world of web development, making the footer stick to the bottom can be a real head-scratcher. The challenge arises when the content on your page doesn’t fill the entire height of the viewport, leaving the footer stranded somewhere in the middle. But worry not, as we’re about to tackle this issue step by step.
Table of Contents
Use Flexbox on your CSS
Flexbox is a layout model that comes to the rescue in many CSS layout challenges, and making the footer stick to the bottom is no exception. In your React component’s stylesheet, wrap your entire content inside a container div and set its display property to ‘flex.’ This allows you to distribute the space evenly between the container and the footer.
// styles.css .container { display: flex; flex-direction: column; min-height: 100vh; /* Ensures the container takes up at least the full height of the viewport */ } .content { flex: 1; /* Allows the content to take up available space, pushing the footer to the bottom */ } .footer { background-color: green; border-top: 2px solid red; position: fixed; width: 100%; bottom: 0; color: white; font-size: 25px; }
Add the Magic Touch to Your React Component
Now that your stylesheet is flexing its muscles, it’s time to integrate it into your React component.
// App.js import React from 'react'; import './styles.css'; const App = () => { return ( <div className="container"> <div className="content"> {/* Your main content goes here */} </div> <div className="footer"> {/* Your footer content goes here */} </div> </div> ); }; export default App;
With these simple yet powerful steps, you’ve conquered the challenge of making your footer stick to the bottom in React. Your website now boasts a professional, polished look, with the footer gracefully anchored at the bottom, regardless of the content’s height.
- Also Read: How to Loop Through Objects in JavaScript?
Conclusion
Mastering the art of making footers stick to the bottom in React involves embracing the flexibility of Flexbox and incorporating it into your component’s styling. By following these steps, you’ve unlocked a valuable skill that adds a touch of finesse to your web development repertoire. So go ahead, implement these techniques, and watch your website’s design reach new heights!