Swapping of two numbers in Python: Hey Folks! In this tutorial you will learn the various methods to swap two number in python. We will discuss many ways to swap two numbers in python that will help you broader your thoughts about programming and this will help you grow into programming more.
Let’s first understand the concept of swapping two numbers.
Let’s suppose we have two numbers N1 and N2 stored in two variables a and b. So before swapping the condition will be like below:
//Before Swapping a = N1 b = N2
Now if we want that N1 should stored in b instead of a similarly N2 should stored in a instead of b, then this process is called swapping of two numbers and the condition will look like below:
//After Swapping a = N2 b = N1
Now lets understand various methods to implement swapping of two numbers in python.
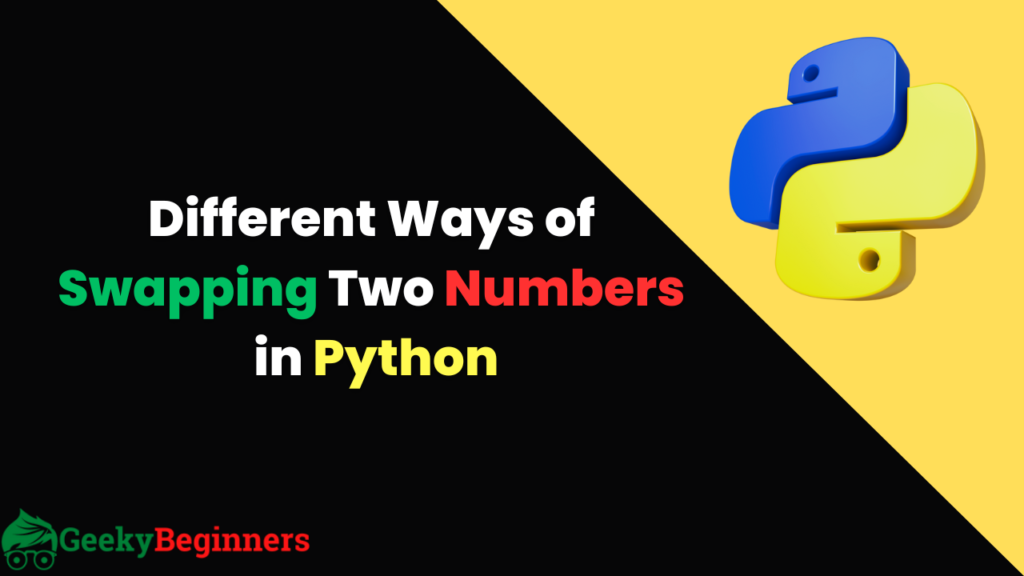
Table of Contents
Swapping of Two Numbers in python using temporary variable
Write a python program to swap two numbers using a third variable.
Steps to write a program
- First of all Print a message ‘Number before swapping: 10, 20
- Store values 10 & 20 in the variables a & b respectively
- Also, assign a to the temporary variable temp
- Now, assign to the b in the a
- Also, assign temp to the b
- Finally, print the swap values of a & b
Source Code
# Swapping of Two Numbers using a Temporary Variable a = 10 b = 20 print("Numbers before swapping: ") print("a =", a) print("b =", b) temp = a a = b b = temp print("Numbers after swapping: ") print("a =", a) print("b =", b)
Swapping of Two Numbers in python without using temporary variable
Write a python program to swap two numbers without using temporary variable.
Steps to Write a Program
- First of all Print a message ‘Number before swapping: 10, 20
- Store values 10 & 20 in the variables a & b respectively.
- Add both variables a & b and then assign to the a.
- apply if condition a>b. if a is greater than b then write the following steps within the if a block of statement.
- subtract b from a and assign to the b
- Also, subtract a from b and assign it to a.
- apply if condition b>a. if b is greater than a then write the following steps within the if a block of statement.
- subtract b from a and assign to the b
- Also, subtract b from a and assign it to a.
- Print both swapped numbers using print() funtion.
Source Code
# Swapping of Two Numbers in python without using temporary variable a = 25 b = 30 print("Numbers before swapping: ") print("a =", a) print("b =", b) a = a + b if a > b: b = a - b a = a - b if b > a: b = b - a a = b - a print("Numbers after swapping: ") print("a =", a) print("b =", b)
Swapping of Two Numbers in python using arithmetic operators
Write a python program to swap two numbers using arithmetic operators.
Steps to Write a Program
- First of all Print a message ‘Number before swapping: 10, 20
- Store values 10 & 20 in the variables a & b respectively.
- Add both variables a & b and then assign to the a.
- Subtract both the variable i.e (a-b) and assign to b.
- Subtract both the variable i.e (a-b) and assign to a.
- Print the swapped values using print() function.
Source Code
# Swapping of Two Numbers in python using arithmetic operators a = 10 b = 20 print("Numbers before swapping: ") print("a =", a) print("b =", b) a = a + b b = a - b a = a - b print("Numbers after swapping: ") print("a =", a) print("b =", b)
Swapping of Two Numbers in python using function
Write a python program to swap two numbers without using function.
Steps to Write a Program
- Print the numbers before swapping 10 & 20.
- declare a function swapNumbers() and implement the following steps within it
- assign a to the temporary variable temp
- Now, assign to the b in the a
- Also, assign temp to the b
- At last, print the message after swapping
- Call the created function swapNumbers()
Source Code
# Swapping of Two Numbers in python using function def swapNumbers(a, b): temp = a a = b b = temp a = 10 b = 20 print("Numbers before swapping: ") print("a =", a) print("b =", b) swapNumbers(a,b) print("Numbers after swapping: ") print("a =", a) print("b =", b)
Swapping of Two Numbers in python using XOR Operator
Write a python program to swap two numbers without using XOR(^) Operator.
Steps to Write a Program
- First of all Print a message ‘Number before swapping: 10, 20
- Store values 10 & 20 in the variables a & b respectively.
- XOR both variables a & b and then assign to the a.
- XOR both variables a & b and then assign to the b.
- Again, XOR both variables a & b and then assign to the a.
- Now print the swapped values using print() function.
Source Code
# Swapping of Two Numbers in python using XOR Operator a = 10 b = 20 print("Numbers before swapping: ") print("a =", a) print("b =", b) a = a ^ b b = a ^ b a = a ^ b print("Numbers after swapping: ") print("a =", a) print("b =", b)
Conclusion
These are the most common ways to swap two numbers in python. We will continuously update this post with more other ways. If you have any doubt or suggestion let us know in the comments section. Happy coding 🙂 !!!